Category >
JQUERY
|| Published on :
Tuesday, July 22, 2014 || Views:
9046
||
javascript examples javascript tutorials learning javascript javascript code
Get QueryString values using JavaScript
For reading QueryString in JavaScript. We will create a name value collection and then retrieving the specific key from that array or collection. For that first create a JavaScript function as below to read querystring in javascript. This Trick wil help the developers in real world conditions
function getQueryStrings() {
//Holds key:value pairs
var queryString = null;
//Get querystring from url
var requestUrl = window.location.search.toString();
if (requestUrl != '') {
//window.location.search returns the part of the URL
//that follows the ? symbol, including the ? symbol
requestUrl = requestUrl.substring(1);
queryString = new Array();
//Get key:value pairs from querystring
var Pairs = requestUrl.split('&');
for (var i = 0; i < Pairs.length; i++) {
var Pair = Pairs[i].split('=');
queryString[Pair[0]] = Pair[1];
}
}
return queryString;
}
Consider an example URL that reads www.sourcecodehub.com.com/file.htm?Q1=123&Q2=345 Next JavaScript function will read the array using the name of the QueryString as below
1 function myfunction() {
2 var queryString = getQueryStrings();
3
4 //if nothing found in querystring
5 if (queryString == null) {
6 alert('no querystring found');
7 return;
8 }
9
10 //URL format: www.sourcecodehub.com.com/file.htm?Q1=123&Q2=345
11 alert(queryString['Q1']);
12 alert(queryString['Q2']);
13 }
Then place input button to see the QueryString values as below
1 "Button1" type="button" value="Click me!!!" onclick="myfunction()" />
Complete Source Codes
1 "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
2
"http://www.w3.org/1999/xhtml"> 34Get QueryString values using JavaScript 5 46 47
48 "Button1" type="button" value="Click me!!!" onclick="myfunction()" /> 49
50
56
5758
Output will be like this....
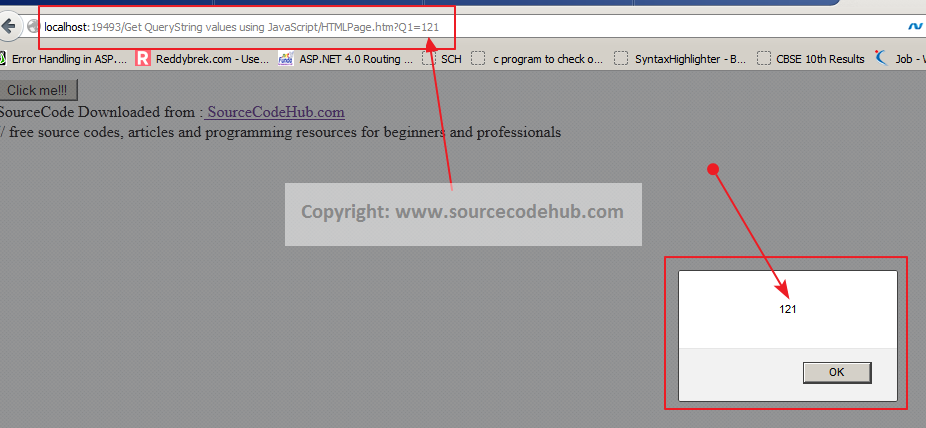
So In this article we learn how we can read QueryString in JavaScript, stay tuned for much and more excited articles on JavaScript.
Download Source Codes